Batch normalization is a technique used in artificial intelligence (AI) and machine learning to standardize the inputs of each layer in a neural network, thereby improving its performance and stability. This technique, often used in conjunction with Python, a popular programming language for AI, has become a staple in the field due to its effectiveness in accelerating the training process of deep neural networks.
Despite its widespread use, the concept of batch normalization can be complex to understand, especially for those new to AI or Python programming. This glossary entry seeks to demystify batch normalization, exploring its purpose, how it works, and how it can be implemented in Python for AI applications.
Understanding Batch Normalization
Batch normalization, introduced by Sergey Ioffe and Christian Szegedy in 2015, is a method used to make artificial neural networks faster and more stable through normalization of the layers’ inputs by re-centering and re-scaling. The name ‘batch’ comes from the way the technique calculates the mean and variance for normalization during training; it uses the current mini-batch of inputs, rather than the entire data set.
Before delving into the specifics of batch normalization, it’s crucial to understand the problem it solves: Internal Covariate Shift. This is a change in the distribution of network activations due to the change in network parameters during training. This shift can slow down the training process and make it harder for the network to converge.
The Importance of Normalization
Normalization is a statistical technique that adjusts the values measured on different scales to a common scale. In the context of neural networks, normalization helps in faster learning and better overall performance. It does so by ensuring that each input parameter (pixel, in the case of images) has a similar data distribution, which makes the learning process more efficient.
Without normalization, the network would be much more difficult to train. This is because the gradients would either vanish (become too small) or explode (become too large), leading to longer training times or causing the network to fail entirely.
How Batch Normalization Works
Batch normalization applies a transformation that maintains the mean output close to 0 and the output standard deviation close to 1. It achieves this by computing the empirical mean and variance independently for each dimension (input node) and using these to normalize the inputs. This operation allows the network to learn more complex patterns and improves overall performance.
Batch normalization also has a beneficial effect on the gradient flow through the network, by reducing the dependence of gradients on the scale of the parameters or of their initial values. This, in turn, makes the network’s training more stable.
Implementing Batch Normalization in Python
Python, with its robust libraries like TensorFlow and Keras, provides an easy-to-use platform for implementing batch normalization. These libraries offer built-in functions for batch normalization, making it accessible even to those with a basic understanding of Python programming.
Let’s look at a simple example of how to implement batch normalization in Python using the Keras library. In this example, we’ll use batch normalization in a simple neural network model for binary classification.
Python Code Example
from keras.models import Sequential
from keras.layers import Dense, BatchNormalization
# define model
model = Sequential()
model.add(Dense(50, input_dim=2, activation='relu', kernel_initializer='he_uniform'))
model.add(BatchNormalization())
model.add(Dense(1, activation='sigmoid'))
# compile model
model.compile(loss='binary_crossentropy', optimizer='adam')
In this code snippet, we first import the necessary modules from Keras. We then define a Sequential model and add a Dense layer with 50 nodes, using a ‘relu’ activation function and ‘he_uniform’ kernel initializer. Following this, we add a BatchNormalization layer. Finally, we add an output Dense layer with a ‘sigmoid’ activation function and compile the model with ‘binary_crossentropy’ loss function and ‘adam’ optimizer.
Understanding the Code
The BatchNormalization layer in Keras normalizes the activations of the previous layer at each batch, i.e., applies a transformation that maintains the mean activation close to 0 and the activation standard deviation close to 1. It is added to the model just after the Dense layer and before the activation function.
The ‘relu’ activation function is used to add non-linearity to the network. The ‘he_uniform’ initializer is used to initialize the weights in a way that keeps the variance of the weights close to zero. The ‘sigmoid’ activation function is used in the output layer to ensure the output values are between 0 and 1, making them suitable for binary classification.
Benefits of Batch Normalization
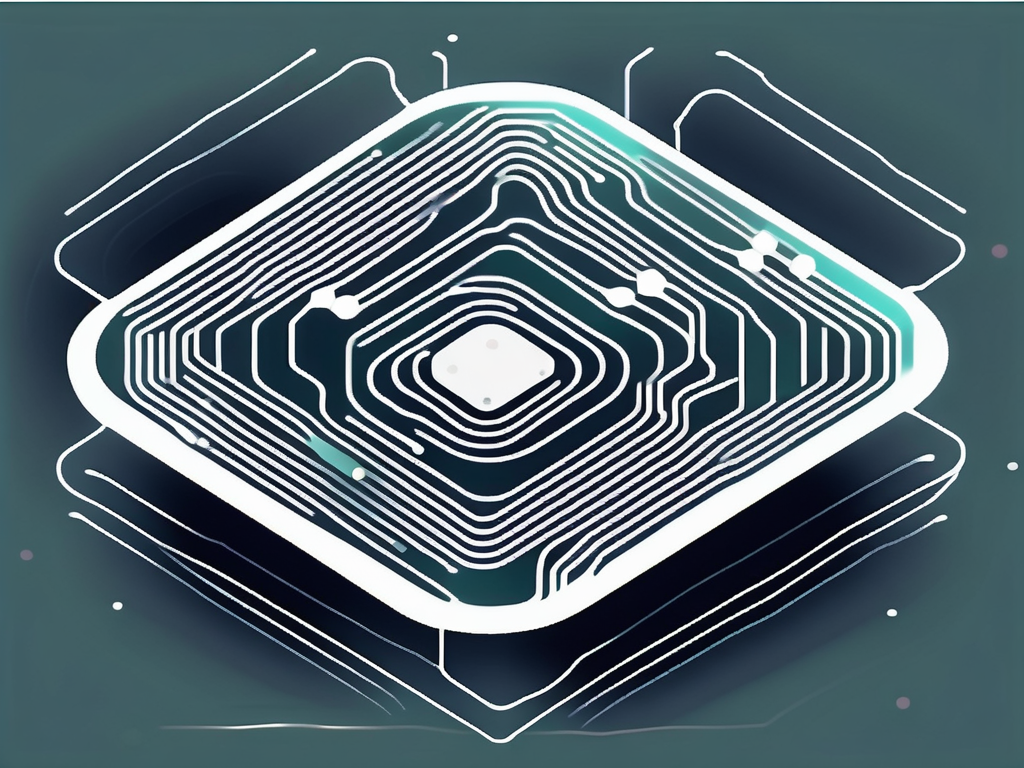
Batch normalization offers several benefits in the context of training deep neural networks. One of the most significant advantages is that it allows for much higher learning rates, accelerating the learning process. This is because the careful normalization of inputs can prevent the learning process from getting stuck in the early stages.
Another benefit of batch normalization is that it makes weights easier to initialize and can make activation functions work better. This is because batch normalization regulates the inputs to be within a certain range, reducing the chance of the activation function getting stuck in the saturated state.
Reducing Overfitting
Batch normalization can also have a slight regularizing effect, reducing the risk of overfitting. This is because the noise introduced by the normalization process adds a bit of randomness to the network’s learning process, similar to dropout. However, this should not be relied upon as a primary method of regularization; it’s more of a nice bonus.
Moreover, batch normalization allows us to use saturating nonlinearities by ensuring that the activation function doesn’t get stuck in the saturated mode, thus improving the performance of the network.
Looking for more inspiration đź“–
- Sam Altman’s secrets on how to generate ideas for any business
- 6 Greg Brockman Secrets on How to Learn Anything – blog
- The secrets behind the success of Mira Murati – finally revealed
- Ideas to Make Money with ChatGPT (with prompts)
- Ilya Sutskever and his AI passion: 7 Deep Dives into his mind
- The “secret” Sam Altman blog post that will change your life
- 4 Life-Changing Lessons we can learn from John McCarthy – one of AI’s Founding Fathers
- Decoding Elon Musk: 5 Insights Into His Personality Type Through Real-Life Examples
Improving Network Training
Batch normalization also helps to make the training of deep networks more manageable. It reduces the sensitivity to the initial starting weights, provides some regularization and noise robustness, and speeds up training, allowing for higher learning rates.
By normalizing activations throughout the network, it mitigates the problem of internal covariate shift, where the distribution of each layer’s inputs changes during training, as parameters of the previous layers change. This leads to faster training and significantly less sensitivity to the initialization of the network.
Limitations of Batch Normalization
Despite its numerous benefits, batch normalization is not without its limitations. One of the main drawbacks is that it adds computational complexity to the model. The need to calculate means and variances for each mini-batch and to apply the normalization operation at each layer can slow down computation, particularly during the training phase.
Another limitation is that batch normalization might not be suitable for all types of neural network architectures. For instance, in recurrent neural networks (RNNs), applying batch normalization is not straightforward due to the temporal dynamics of RNNs.
Dependency on Batch Size
Batch normalization depends on the batch size used during training. Small batch sizes can lead to inaccurate estimates of the mean and variance, leading to unstable training. On the other hand, larger batch sizes can lead to longer training times and higher memory requirements.
Moreover, batch normalization is less effective in online learning models and models with small batch sizes. This is because the estimates of the mean and variance become less accurate as the batch size decreases.
Difficulty with RNNs
As mentioned earlier, applying batch normalization to recurrent neural networks (RNNs) is not straightforward. This is because RNNs have a temporal dimension, meaning that the statistics need to be calculated along this dimension, which can be challenging.
Furthermore, batch normalization can potentially introduce a discrepancy between the training and inference stages. During training, the mean and variance are calculated for each mini-batch, but during inference, an average of these statistics is used. This discrepancy can sometimes lead to performance degradation.
Conclusion
Batch normalization is a powerful technique in the field of deep learning, offering numerous benefits such as faster training times, less sensitivity to initialization, and the ability to use higher learning rates. Implemented in Python using libraries like TensorFlow and Keras, it has become an essential tool in the toolbox of any AI developer.
Despite its limitations, such as added computational complexity and challenges with certain types of networks, the benefits of batch normalization often outweigh its drawbacks, making it a widely used technique in the field of AI and machine learning.