Optimization algorithms are a set of procedures that are used to modify a system in order to make it work more efficiently or use fewer resources. In the context of Python for Artificial Intelligence (AI), these algorithms are used to optimize the performance of AI models, making them faster, more accurate, and more efficient. This article delves into the intricacies of optimization algorithms, their role in AI, and how they are implemented using Python.
Python is a versatile and powerful programming language that is widely used in the field of AI. Its simplicity and readability make it an ideal choice for implementing complex AI models and algorithms. Moreover, Python’s extensive library support, including libraries like NumPy, SciPy, and TensorFlow, makes it even more suitable for AI development.
Understanding Optimization Algorithms
Optimization algorithms are mathematical tools used for finding the best solution or minimizing/maximizing a function. They are used in various fields, including physics, engineering, economics, and computer science. In AI, optimization algorithms play a crucial role in training machine learning models by minimizing the error or loss function.
Optimization algorithms can be broadly classified into two types: deterministic and stochastic. Deterministic algorithms, such as Gradient Descent, use specific rules and deterministic models to find the optimal solution. On the other hand, stochastic algorithms, like Genetic Algorithm, use randomness in their search process.
Role of Optimization Algorithms in AI
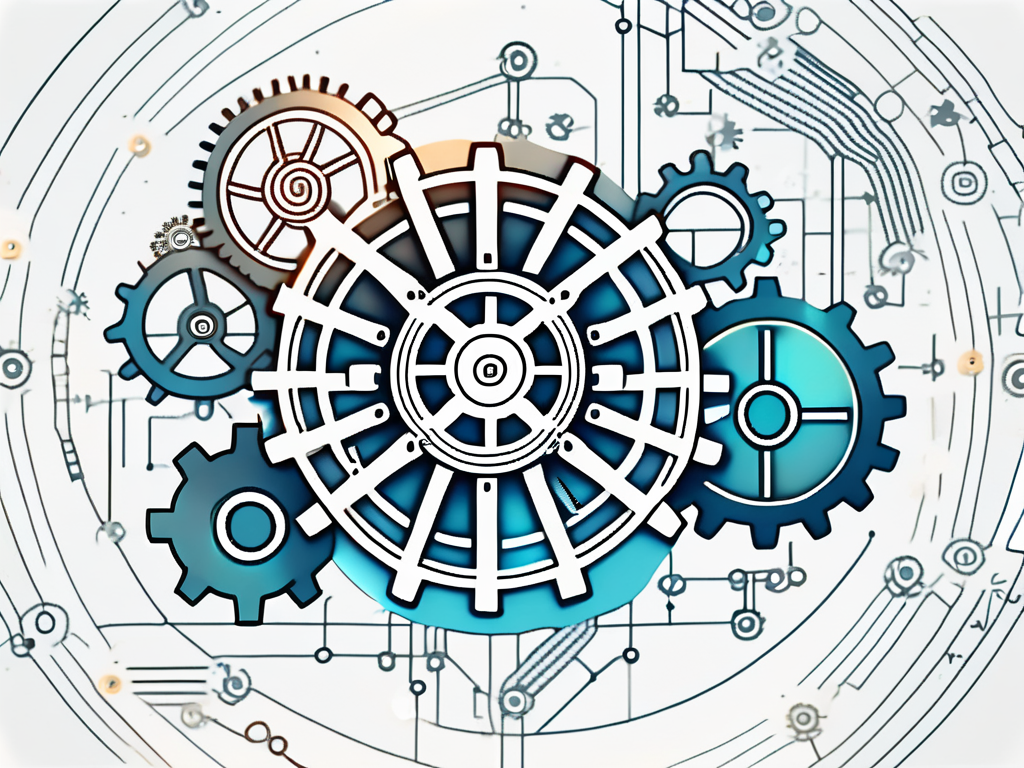
In AI, optimization algorithms are used to optimize the parameters of a model to improve its performance. This is done by minimizing the loss function, which is a measure of the difference between the model’s predictions and the actual data. The optimization algorithm iteratively adjusts the model’s parameters to find the minimum of the loss function.
Optimization algorithms also play a crucial role in deep learning, a subset of machine learning that involves neural networks with many layers. These algorithms are used to adjust the weights and biases of the neural networks to minimize the error in the output.
Types of Optimization Algorithms
There are several types of optimization algorithms used in AI, each with its own strengths and weaknesses. Some of the most commonly used algorithms include Gradient Descent, Stochastic Gradient Descent, Mini-Batch Gradient Descent, Momentum, RMSprop, and Adam.
Gradient Descent is the most basic type of optimization algorithm. It uses the gradient of the loss function to find the direction of the steepest descent and adjusts the parameters accordingly. Stochastic Gradient Descent, on the other hand, updates the parameters using a single training example at a time, making it faster but less stable than Gradient Descent.
Implementing Optimization Algorithms in Python
Python, with its extensive library support, provides several ways to implement optimization algorithms. Libraries like NumPy and SciPy provide basic functions for numerical computation, while TensorFlow and PyTorch provide advanced features for implementing and training AI models.
Let’s take a look at how to implement a basic Gradient Descent algorithm in Python using NumPy. First, we need to define the function we want to optimize and its gradient. Then, we initialize the parameters and iteratively update them using the gradient of the function.
Example: Gradient Descent in Python
Here’s a simple example of how to implement the Gradient Descent algorithm in Python:
import numpy as np # Function to optimize def f(x): return x**2 # Gradient of the function def df(x): return 2*x # Gradient Descent def gradient_descent(x_start, learning_rate, n_iterations): x = x_start for i in range(n_iterations): x -= learning_rate * df(x) return x # Test the function x_start = 5 learning_rate = 0.1 n_iterations = 100 x_opt = gradient_descent(x_start, learning_rate, n_iterations) print('Optimal x:', x_opt)
In this example, we’re trying to find the minimum of the function f(x) = x^2. We start at x = 5 and update x using the gradient of the function, which is 2*x. After 100 iterations, we find that the optimal x is close to 0, which is the expected result.
Advanced Optimization Algorithms in Python
While Gradient Descent is a simple and effective optimization algorithm, it has some limitations. For example, it can get stuck in local minima and is sensitive to the learning rate. To overcome these limitations, several advanced optimization algorithms have been developed.
These advanced algorithms, such as Adam, RMSprop, and AdaGrad, use adaptive learning rates to speed up convergence and avoid getting stuck in local minima. They also incorporate momentum to avoid oscillations and speed up the learning process.
Example: Adam Optimizer in Python
The Adam optimizer is a popular optimization algorithm used in deep learning. It combines the advantages of two other extensions of stochastic gradient descent: AdaGrad and RMSProp. Here’s how to implement the Adam optimizer in Python using TensorFlow:
import tensorflow as tf # Create a simple model model = tf.keras.models.Sequential([ tf.keras.layers.Dense(10, activation='relu', input_shape=(32,)), tf.keras.layers.Dense(1) ]) # Compile the model with the Adam optimizer model.compile(optimizer='adam', loss='mean_squared_error')
In this example, we’re creating a simple neural network with one hidden layer and one output layer. We then compile the model using the Adam optimizer and the mean squared error loss function. This sets up the model for training, which can be done using the model.fit() function.
Looking for inspiration đź“–
- Sam Altman’s secrets on how to generate ideas for any business
- 6 Greg Brockman Secrets on How to Learn Anything – blog
- The secrets behind the success of Mira Murati – finally revealed
- Ideas to Make Money with ChatGPT (with prompts)
- Ilya Sutskever and his AI passion: 7 Deep Dives into his mind
- The “secret” Sam Altman blog post that will change your life
- 4 Life-Changing Lessons we can learn from John McCarthy – one of AI’s Founding Fathers
- Decoding Elon Musk: 5 Insights Into His Personality Type Through Real-Life Examples
Conclusion
Optimization algorithms are a crucial part of AI, as they are used to train and optimize AI models. Python, with its simplicity and extensive library support, is an ideal language for implementing these algorithms. Whether you’re using basic algorithms like Gradient Descent or advanced ones like Adam, Python provides all the tools you need to implement and optimize your AI models.
As AI continues to evolve, so too will the optimization algorithms used to train AI models. By understanding these algorithms and how to implement them in Python, you can stay at the forefront of this exciting field.